How to Create a Chat App in Android: A Comprehensive Guide
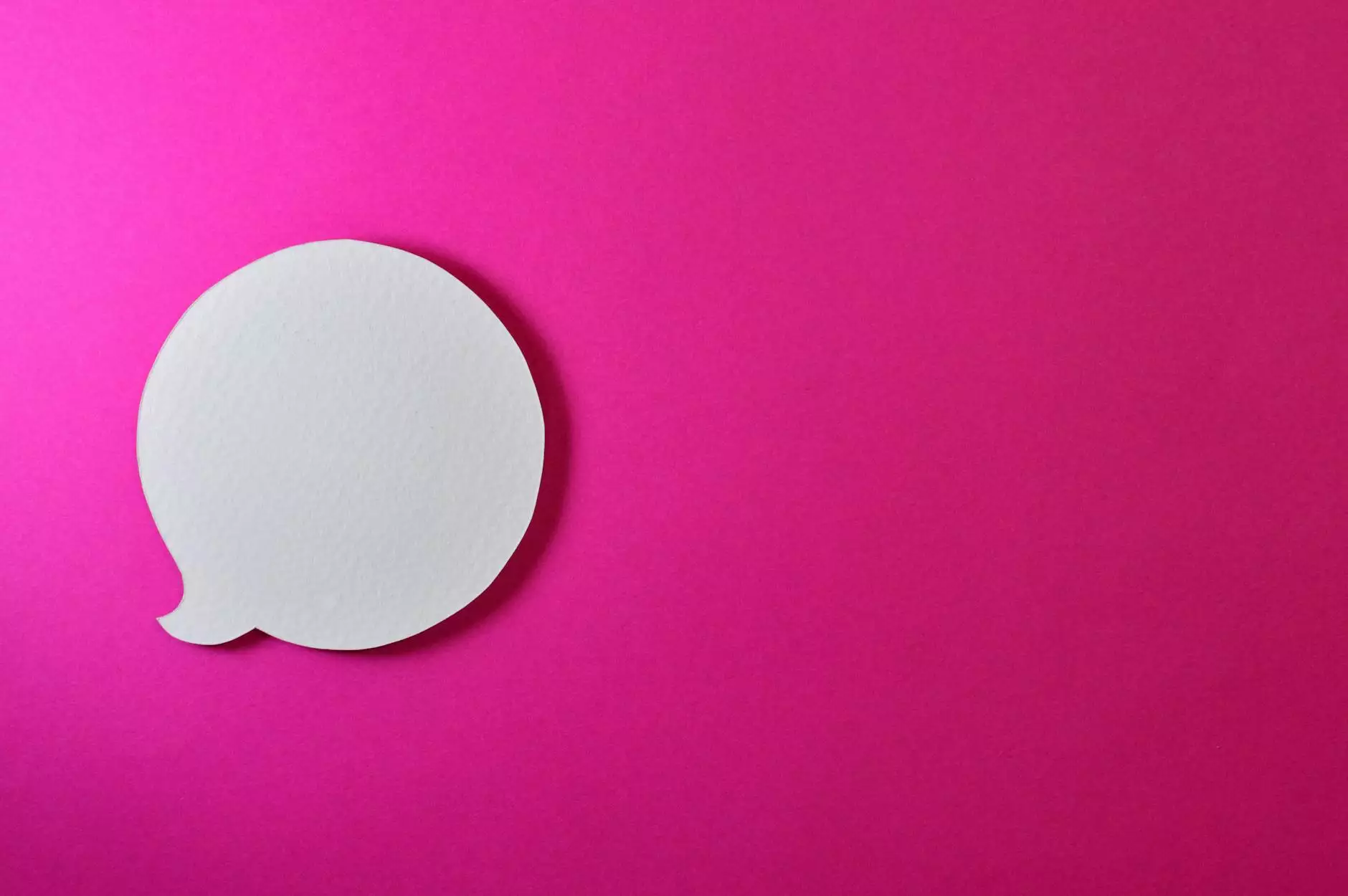
In today's digital age, communication has become a vital part of our lives. With the rise of mobile applications, creating a chat app has never been more desirable. This guide will serve as your ultimate resource on how to create a chat app in Android, ensuring you understand each necessary step, from planning to deployment.
Understanding the Basics of Chat Applications
Before diving into the technical aspects of development, it is essential to understand what a chat application entails. At its core, a chat app enables users to send and receive messages in real-time. Here are some critical features to consider:
- User Registration: Allow users to create accounts efficiently.
- Real-Time Messaging: Implement technologies that facilitate instant communication.
- Push Notifications: Keep users informed about new messages.
- Media Sharing: Enable users to send images, videos, and other files.
- Group Chats: Allow multiple users to communicate within a single thread.
Essential Tools and Technologies
When it comes to creating a chat app in Android, having the right tools at your disposal is crucial. Below are some essential tools and technologies you'll need:
- Android Studio: The official IDE for Android development, perfect for building robust apps.
- Firebase: A powerful platform that provides real-time database services and authentication.
- WebSocket: A protocol for full-duplex communication channels over a single TCP connection, crucial for real-time messaging.
- Retrofit: A type-safe HTTP client for Android and Java, making it easier to interact with APIs.
- Glide: A powerful image loading and caching library for Android.
Step-by-Step Guide to Create Your Chat App
Step 1: Planning Your Chat Application
Before any code is written, it is vital to plan your app's structure. Ask yourself these questions:
- What is the app’s primary goal?
- Who is the target audience?
- What features are essential for MVP (Minimum Viable Product)?
Step 2: Setting Up Android Studio
Once your planning phase is complete, it’s time to set up your development environment:
- Download and install Android Studio.
- Open Android Studio and create a new project.
- Choose an appropriate name and package for your app.
Step 3: Implementing User Registration and Authentication
For your app to be functional, you need an efficient user registration system. Using Firebase Authentication will streamline this process:
- Go to the Firebase Console and create a new project.
- Enable Email/Password authentication from the Authentication section.
- Integrate Firebase SDK into your Android project.
- Design the UI for registration and login screens using XML layout files.
- Write code to handle registration and login using FirebaseAuth.
Step 4: Setting Up Real-Time Database
A chat app requires a database to store messages. Firebase Realtime Database is an excellent choice:
- In the Firebase Console, navigate to the Realtime Database section and create a new database.
- Set rules for your database to allow read and write access for authenticated users.
- Create a data structure in JSON format, such as: { "users": { "userId1": { "name": "User One", "lastSeen": "timestamp" }, "userId2": { "name": "User Two", "lastSeen": "timestamp" } }, "messages": { "chatId": { "messageId1": { "senderId": "userId1", "text": "Hello!", "timestamp": "timestamp" } } } }
Step 5: Implementing Messaging Functionality
With your database set, you can now implement real-time messaging:
- Use Firebase Database references to add messages to the database.
- Implement a listener to retrieve messages from the database: DatabaseReference messagesRef = FirebaseDatabase.getInstance().getReference("messages/chatId"); messagesRef.addValueEventListener(new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { for (DataSnapshot messageSnapshot: dataSnapshot.getChildren()) { String message = messageSnapshot.child("text").getValue(String.class); // Update UI with the new message } } @Override public void onCancelled(DatabaseError databaseError) { // Handle any errors } });
Step 6: Enabling Push Notifications
To notify users of incoming messages, integrating Firebase Cloud Messaging (FCM) is essential:
- Set up FCM in the Firebase Console.
- Implement FCM in your Android app by adding the necessary dependencies in your Gradle files.
- Create a service to handle incoming notifications: public class MyFirebaseMessagingService extends FirebaseMessagingService { @Override public void onMessageReceived(RemoteMessage remoteMessage) { // Handle the message and show a notification } }
Step 7: Designing the User Interface
The user experience significantly impacts the success of your chat app. Use Material Design principles to create an attractive UI:
- Activity Layouts: Design different XML layouts for various activities, such as chat lists and conversations.
- RecyclerView: Use RecyclerView to display messages dynamically and efficiently.
- Animations: Add smooth transitions and animations to enhance user interaction.
Step 8: Testing Your Application
Thorough testing is crucial to ensure your app works seamlessly:
- Unit Testing: Test specific components of your application to ensure they work as intended.
- Integration Testing: Verify that different parts of your app function cohesively.
- User Acceptance Testing: Conduct beta testing with real users for feedback.
Step 9: Deploying Your Chat Application
After successful testing, you are ready to launch your app:
- Prepare your app for release by generating a signed APK in Android Studio.
- Publish your app on the Google Play Store and provide a detailed app description.
- Market your app through social media and digital marketing campaigns to increase visibility.
Best Practices for Developing a Chat App
Creating a successful chat app involves adhering to the best practices in software development. Here are some crucial guidelines:
- Prioritize Security: Implement strong encryption for messages to protect user data.
- Monitor Performance: Continuously optimize your app’s performance for a smooth user experience.
- User Privacy: Respect user privacy by not collecting unnecessary data and providing clear privacy policies.
- Regular Updates: Keep your application updated with the latest features and security improvements.
Conclusion
In conclusion, developing a chat application in Android is an exciting endeavor that combines creativity, technical skills, and a deep understanding of user needs. By following this comprehensive guide on how to create a chat app in Android, you’re well on your way to building an app that can compete in today’s vibrant marketplace. Always remember that feedback is essential, so involve your users during the development process and iterate on their suggestions to refine your application.
With dedication and careful planning, your chat app can become a reliable platform for communication, reaching users globally and fostering connections. Happy coding!